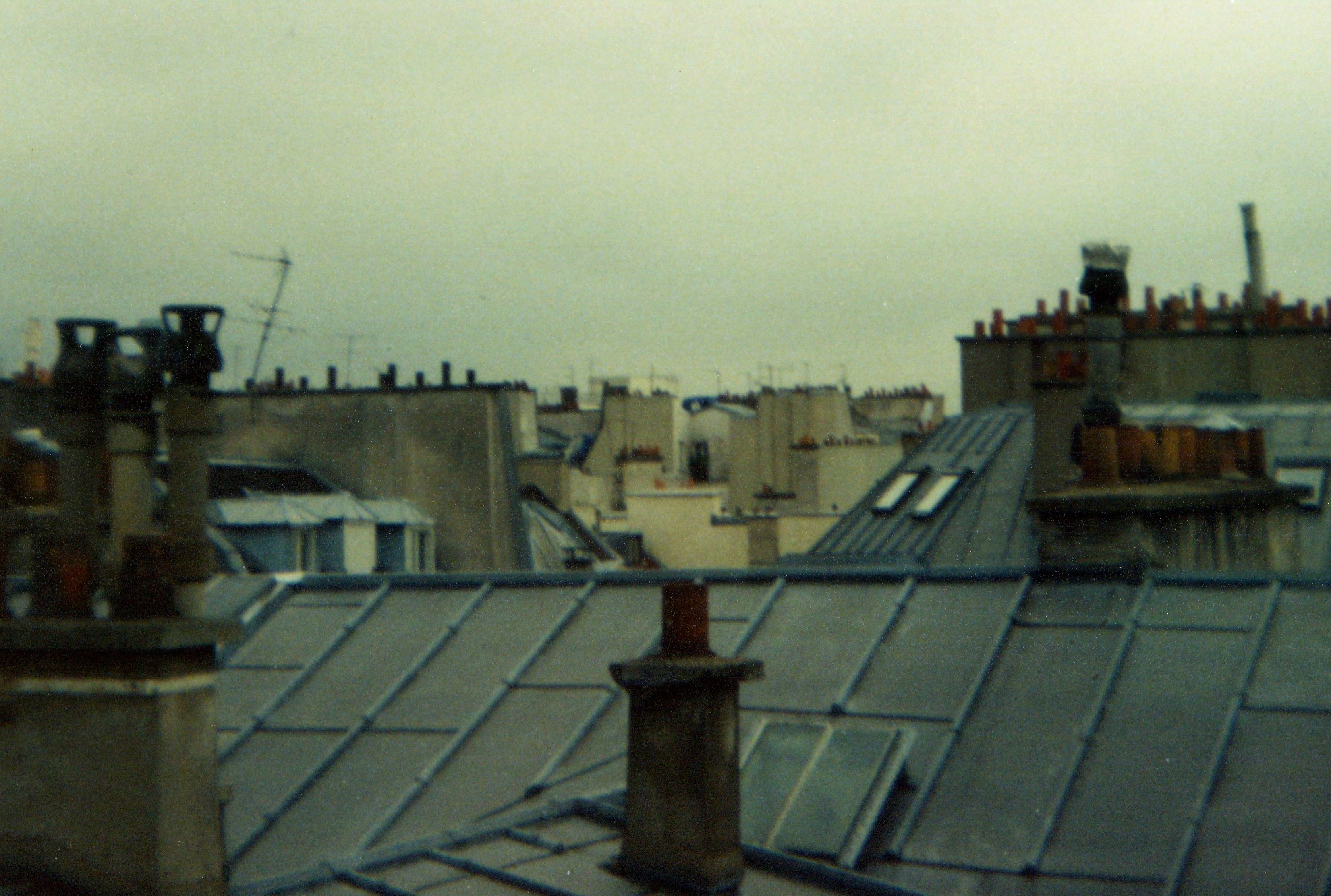
Arrays and Hashes
Arrays
Arrays are ordered collections which can contain any Object type including Integers, Strings, and even other Arrays or Hashses.
For example, x = [1, 2, 3, 4]
is an Array containing four elements.
Arrays are Integer Indexed starting at 0 so our four element Array has the following Indexes:
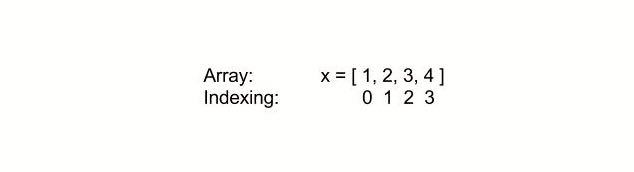
Elements from within the Array can be accessed using the #[]
Method so as an example: x[2] #=> 3
Once created, there are many Array Methods that can be used to:
- Obtain information about the Array
- Add elements to the Array
- Remove elements from the Array
- Iterate over the Array
- Select items from the Array
For more information on the various Array Methods available, check out the API on Arrays at http://ruby-doc.org/core-2.2.0/Array.html
Hashes
Like Arrays, Hashes are also a collection of Objects. The difference is in the storage format. Instead of using Indexed Positioning, Hashes use Keys to identify Values. Both Keys and Values can use any Object type. Hashes are often referred to as being dictionary-like because Values can be looked up using their Keys.
Here's a basic Hash:
concert_tshirt_quantity = { s: 14, m: 12, l: 28, xl: 17 }
Similar to to Arrays, information in our Hash can be accessed using the < code>#[] method. For example: concert_tshirt_quantity[:m] #=> 12
One of the advantages of using Hashes over Arrays is that they are not order dependent and that keys themselves can have meaning as opposed to Array indexing which only represents positioning.
Like Arrays, Hashes also have many methods available. For more information on the various Hash Methods available, check out the API on Hashes at http:// ruby-doc.org/core-2.2.3/Hash.html
Both Arrays and Hashes can utilize the Enumerable module to take advantage of methods. More information can be found at http://ruby-doc.org/core-2.2.3 /Enumerable.html