Regular Expressions
Every so often while solving Ruby exercises, I come across situations where I need to evaluate strings. The Ruby String API has lots of methods to choose from but as I start building out logic to iterate through characters, I remember - Regular Expressions.
A regular expression (or RegEx) is basically string pattern matching. You construct a pattern and use it to compare against strings to determine if that pattern exists within the string.
Regular expressions are simple and powerful. Before diving into some of their more complex capabilities, let's start with a basic example to show the syntax.
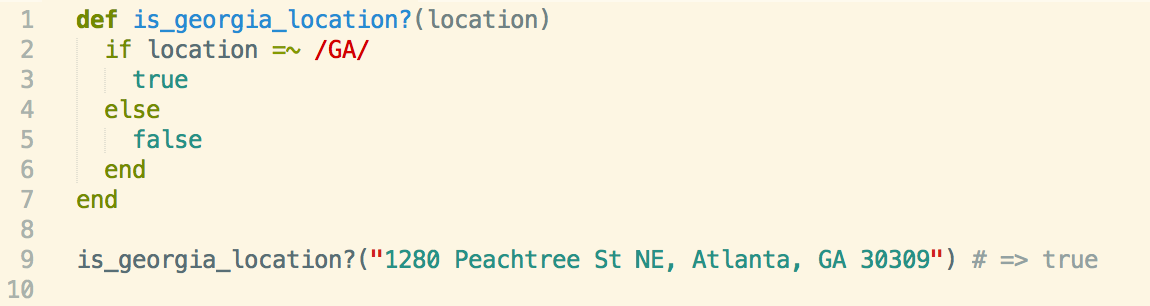
In this example, the location argument is compared (using =~) to the RegEx statement which appears between the two forward slashes. Any pattern between those two slashes will be compared against the string and will return true if the pattern is found.
Matching against letters and numbers is as simple as the example above. Punctuation characters have some special meaning so if you want to find matches that include the period punctuation, use a backslash to turn off the special meaning like so:
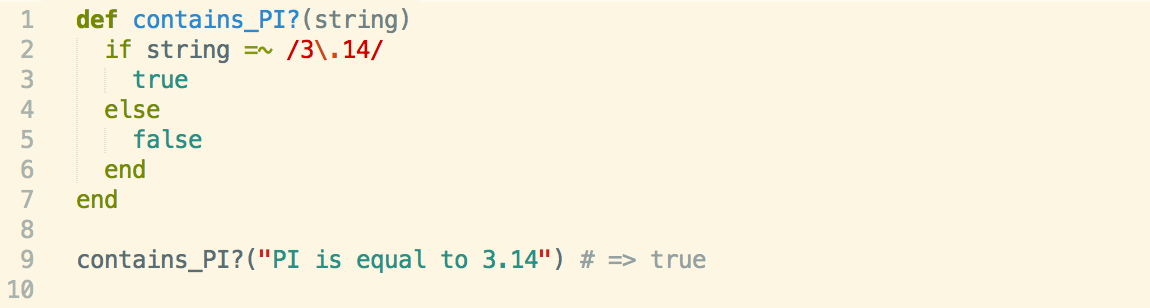
Regular expressions can evaluate against sets as well by including the set between square brackets.
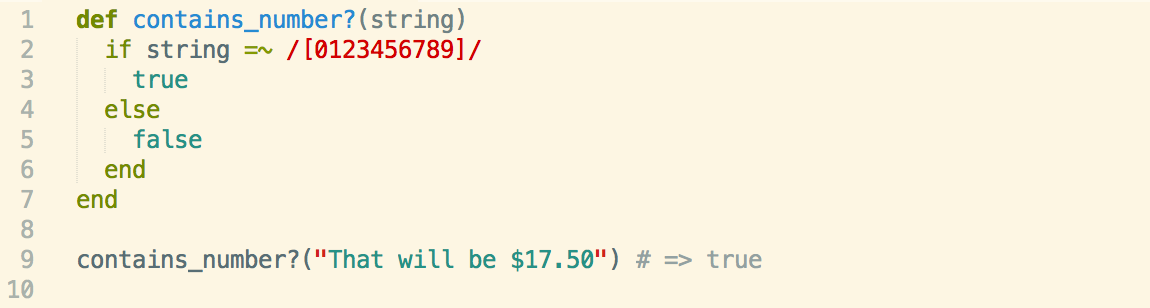
There are shortcuts if writing out each number is too much work. The following three expressions will all evaluate any digit.

There are shortcuts for letters also. Letter evaluation is case sensitive so the first two examples only search for lower case letters. The second two search for any letter regardless of case.

The vertical bar can be used to search for alternatives. The following will return true for any string containing either a train or a plane.

Eloquent Ruby gives a good overview of regular expressions and one of the more complex examples they use is how to search a string for time in the following format: '09:24 AM'. Using what we learned above this could be solved as follows:
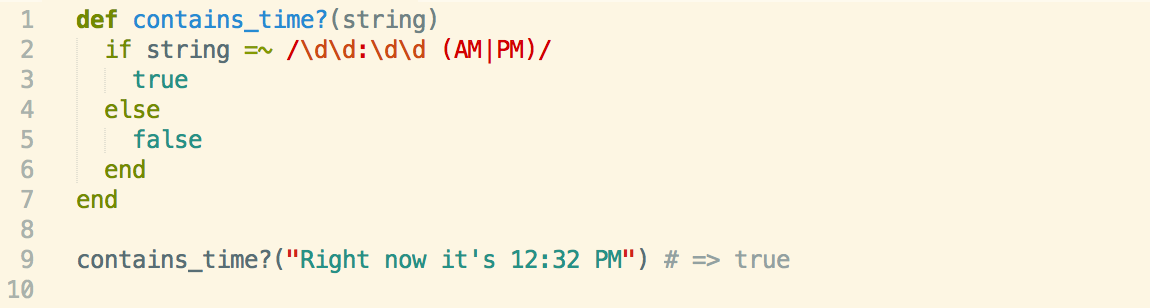
This should provide a good starting point to understand how RegEx is used and give enough understanding to start unpacking some of the more complex solutions. When searching online I'm amazed by how some solutions can be so succinct.
A resource I like to use while building regular expressions is rubular.com This site allows you to build out a RegEx statement and test against strings.